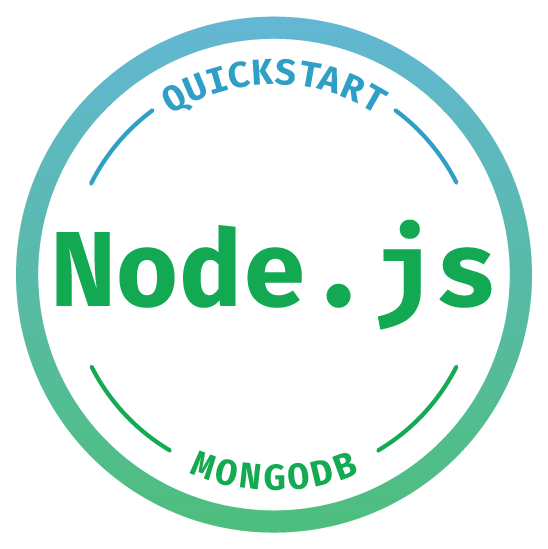
Developers who move from relational databases to MongoDB commonly ask, "Does MongoDB support ACID transactions? If so, how do you create a transaction?" The answer to the first question is, "Yes!"
Beginning in 4.0 MongoDB added support for multi-document ACID transactions, and beginning in 4.2 MongoDB added support for distributed ACID transactions. If you're not familiar with what ACID transactions are or if you should be using them in MongoDB, check out my earlier post on the subject.
For those of you just joining us in this Quick Start with MongoDB and Node.js series, welcome! We began by walking through how to connect to MongoDB and perform each of the CRUD — create, read, update, and delete — operations. Then we jumped into more advanced topics like the aggregation framework.
The code we write today will use the same structure as the code we built in the first post in the series, so, if you have any questions about how to get started or how the code is structured, head back to that first post.
Now let's dive into that second question developers ask--let's discover how to create a transaction!
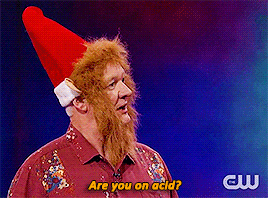
Get started with an M0 cluster on Atlas today. It's free forever, and it's the easiest way to try out the steps in this blog series.
#Creating an Airbnb Reservation
As you may have experienced while working with MongoDB, most use cases do not require you to use multi-document transactions. When you model your data using our rule of thumb Data that is accessed together should be stored together, you'll find that you rarely need to use a multi-document transaction. In fact, I struggled a bit to think of a use case for the Airbnb dataset that would require a multi-document transaction.
After a bit of brainstorming, I came up with a somewhat plausible example. Let's say we want to allow users to create reservations in the sample_airbnb database
.
We could begin by creating a collection named users. We want users to be able to easily view their reservations when they are looking at their profiles, so we will store the reservations as embedded documents in the users collection. For example, let's say a user named Leslie creates two reservations. Her document in the users collection would look like the following:
1 { 2 "_id": {"$oid":"5dd589544f549efc1b0320a5"}, 3 "email": "leslie@example.com", 4 "name": "Leslie Yepp", 5 "reservations": [ 6 { 7 "name":"Infinite Views", 8 "dates": [ 9 {"$date": {"$numberLong":"1577750400000"}}, 10 {"$date": {"$numberLong":"1577836800000"}} 11 ], 12 "pricePerNight": {"$numberInt":"180"}, 13 "specialRequests": "Late checkout", 14 "breakfastIncluded":true 15 }, 16 { 17 "name": "Lovely Loft", 18 "dates": [ 19 {"$date": {"$numberLong": "1585958400000"}} 20 ], 21 "pricePerNight": {"$numberInt":"210"} 22 "breakfastIncluded":false 23 } 24 ] 25 }
When browsing Airbnb listings, users need to know if the listing is already booked for their travel dates. As a result we want to store the dates the listing is reserved in the listingsAndReviews
collection. For example, the "Infinite Views" listing that Leslie reserved should be updated to list her reservation dates.
1 { 2 "_id": {"$oid":"5dbc20f942073d6d4dabd730"}, 3 "name":"Infinite Views", 4 "summary":"Modern home with infinite views from the infinity pool", 5 "property_type": "House", 6 "bedrooms": {"$numberInt": "6"}, 7 "bathrooms":{"$numberDouble":"4.5"}, 8 "beds":{"$numberInt":"8"}, 9 "datesReserved": [ 10 {"$date": {"$numberLong": "1577750400000"}}, 11 {"$date": {"$numberLong": "1577836800000"}} 12 ] 13 }
Keeping these two records in sync is imperative. If we were to create a reservation in a document in the users collection without updating the associated document in the listingsAndReviews
collection, our data would be inconsistent. We can use a multi-document transaction to ensure both updates succeed or fail together.
#Set Up
As with all posts in this MongoDB and Node.js Quick Start series, you'll need to ensure you've completed the prerequisite steps outlined in the Set up section of the first post in this series.
We'll be using the "Infinite Views" Airbnb listing we created in a previous post in this series. Hop back to the post on Creating Documents if your database doesn't currently have the Infinite Views listing.
The Airbnb sample dataset only has the listingsAndReviews collection by default. To help you quickly create the necessary collection and data, I wrote usersCollection.js. Download a copy of the file, update the uri constant to reflect your Atlas connection info, and run it by executing node usersCollection.js
. The script will create three new users in the users collection: Leslie Yepp, April Ludfence, and Tom Haverdodge. If the users collection does not already exist, MongoDB will automatically create it for you when you insert the new users. The script also creates an index on the email
field in the users collection. The index requires that every document in the users collection has a unique email
.
#Create a Transaction in Node.js
Now that we are set up, let's implement the functionality to store Airbnb reservations.
#Get a Copy of the Node.js Template
To make following along with this blog post easier, I've created a starter template for a Node.js script that accesses an Atlas cluster.
- Download a copy of template.js.
- Open template.js in your favorite code editor.
- Update the Connection URI to point to your Atlas cluster. If you're not sure how to do that, refer back to the first post in this series.
- Save the file as
transaction.js
.
You can run this file by executing node transaction.js
in your shell. At this point, the file simply opens and closes a connection to your Atlas cluster, so no output is expected. If you see DeprecationWarnings, you can ignore them for the purposes of this post.
#Create a Helper Function
Let's create a helper function. This function will generate a reservation document that we will use later.
- Paste the following function in
transaction.js
:
1 function createReservationDocument(nameOfListing, reservationDates, reservationDetails) { 2 // Create the reservation 3 let reservation = { 4 name: nameOfListing, 5 dates: reservationDates, 6 } 7 8 // Add additional properties from reservationDetails to the reservation 9 for (let detail in reservationDetails) { 10 reservation[detail] = reservationDetails[detail]; 11 } 12 13 return reservation; 14 }
To give you an idea of what this function is doing, let me show you an example. We could call this function from inside of main()
:
1 createReservationDocument("Infinite Views", 2 [new Date("2019-12-31"), new Date("2020-01-01")], 3 { pricePerNight: 180, specialRequests: "Late checkout", breakfastIncluded: true });
The function would return the following:
1 { 2 name: 'Infinite Views', 3 dates: [ 2019-12-31T00:00:00.000Z, 2020-01-01T00:00:00.000Z ], 4 pricePerNight: 180, 5 specialRequests: 'Late checkout', 6 breakfastIncluded: true 7 }
#Create a Function for the Transaction
Let's create a function whose job is to create the reservation in the database.
- Continuing to work in transaction.js, create an asynchronous function named
createReservation
. The function should accept a MongoClient, the user's email address, the name of the Airbnb listing, the reservation dates, and any other reservation details as parameters.
1 async function createReservation(client, userEmail, nameOfListing, reservationDates, reservationDetails) { 2 }
- Now we need to access the collections we will update in this function. Add the following code to
createReservation()
.
1 const usersCollection = client.db("sample_airbnb").collection("users"); 2 const listingsAndReviewsCollection = client.db("sample_airbnb").collection("listingsAndReviews");
- Let's create our reservation document by calling the helper function we created in the previous section. Paste the following code in
createReservation()
.
1 const reservation = createReservationDocument(nameOfListing, reservationDates, reservationDetails);
- Every transaction and its operations must be associated with a session. Beneath the existing code in
createReservation()
, start a session.
1 const session = client.startSession();
- We can choose to define options for the transaction. We won't get into the details of those here. You can learn more about these options in the driver documentation. Paste the following beneath the existing code in
createReservation()
.
1 const transactionOptions = { 2 readPreference: 'primary', 3 readConcern: { level: 'local' }, 4 writeConcern: { w: 'majority' } 5 };
- Now we're ready to start working with our transaction. Beneath the existing code in
createReservation()
, open atry{}
block, follow it with acatch{}
block, and finish it with afinally{}
block.
1 try { 2 3 } catch(e){ 4 5 } finally { 6 7 }
- We can use ClientSession's withTransaction() to start a transaction, execute a callback function, and commit (or abort on error) the transaction.
withTransaction()
requires us to pass a function that will be run inside the transaction. Add a call towithTransaction()
inside oftry
{}. Let's begin by passing an anonymous asynchronous function towithTransaction()
.
1 const transactionResults = await session.withTransaction(async () => {}, transactionOptions);
- The anonymous callback function we are passing to
withTransaction()
doesn't currently do anything. Let's start to incrementally build the database operations we want to call from inside of that function. We can begin by adding a reservation to the reservations array inside of the appropriate user document. Paste the following inside of the anonymous function that is being passed towithTransaction()
.
1 const usersUpdateResults = await usersCollection.updateOne( 2 { email: userEmail }, 3 { $addToSet: { reservations: reservation } }, 4 { session }); 5 console.log(`${usersUpdateResults.matchedCount} document(s) found in the users collection with the email address ${userEmail}.`); 6 console.log(`${usersUpdateResults.modifiedCount} document(s) was/were updated to include the reservation.`);
- Since we want to make sure that an Airbnb listing is not double-booked for any given date, we should check if the reservation date is already listed in the listing's
datesReserved
array. If so, we should abort the transaction. Aborting the transaction will rollback the update to the user document we made in the previous step. Paste the following beneath the existing code in the anonymous function.
1 const isListingReservedResults = await listingsAndReviewsCollection.findOne( 2 { name: nameOfListing, datesReserved: { $in: reservationDates } }, 3 { session }); 4 if (isListingReservedResults) { 5 await session.abortTransaction(); 6 console.error("This listing is already reserved for at least one of the given dates. The reservation could not be created."); 7 console.error("Any operations that already occurred as part of this transaction will be rolled back."); 8 return; 9 }
- The final thing we want to do inside of our transaction is add the reservation dates to the
datesReserved
array in thelistingsAndReviews
collection. Paste the following beneath the existing code in the anonymous function.
1 const listingsAndReviewsUpdateResults = await listingsAndReviewsCollection.updateOne( 2 { name: nameOfListing }, 3 { $addToSet: { datesReserved: { $each: reservationDates } } }, 4 { session }); 5 console.log(`${listingsAndReviewsUpdateResults.matchedCount} document(s) found in the listingsAndReviews collection with the name ${nameOfListing}.`); 6 console.log(`${listingsAndReviewsUpdateResults.modifiedCount} document(s) was/were updated to include the reservation dates.`);
- We'll want to know if the transaction succeeds. If
transactionResults
is defined, we know the transaction succeeded. IftransactionResults
is undefined, we know that we aborted it intentionally in our code. Beneath the definition of thetransactionResults
constant, paste the following code.
1 if (transactionResults) { 2 console.log("The reservation was successfully created."); 3 } else { 4 console.log("The transaction was intentionally aborted."); 5 }
- Let's log any errors that are thrown. Paste the following inside of
catch(e){ }
:
1 console.log("The transaction was aborted due to an unexpected error: " + e);
- Regardless of what happens, we need to end our session. Paste the following inside of
finally { }
:
1 await session.endSession();
At this point, your function should look like the following:
1 async function createReservation(client, userEmail, nameOfListing, reservationDates, reservationDetails) { 2 3 const usersCollection = client.db("sample_airbnb").collection("users"); 4 const listingsAndReviewsCollection = client.db("sample_airbnb").collection("listingsAndReviews"); 5 6 const reservation = createReservationDocument(nameOfListing, reservationDates, reservationDetails); 7 8 const session = client.startSession(); 9 10 const transactionOptions = { 11 readPreference: 'primary', 12 readConcern: { level: 'local' }, 13 writeConcern: { w: 'majority' } 14 }; 15 16 try { 17 const transactionResults = await session.withTransaction(async () => { 18 19 const usersUpdateResults = await usersCollection.updateOne( 20 { email: userEmail }, 21 { $addToSet: { reservations: reservation } }, 22 { session }); 23 console.log(`${usersUpdateResults.matchedCount} document(s) found in the users collection with the email address ${userEmail}.`); 24 console.log(`${usersUpdateResults.modifiedCount} document(s) was/were updated to include the reservation.`); 25 26 27 const isListingReservedResults = await listingsAndReviewsCollection.findOne( 28 { name: nameOfListing, datesReserved: { $in: reservationDates } }, 29 { session }); 30 if (isListingReservedResults) { 31 await session.abortTransaction(); 32 console.error("This listing is already reserved for at least one of the given dates. The reservation could not be created."); 33 console.error("Any operations that already occurred as part of this transaction will be rolled back."); 34 return; 35 } 36 37 const listingsAndReviewsUpdateResults = await listingsAndReviewsCollection.updateOne( 38 { name: nameOfListing }, 39 { $addToSet: { datesReserved: { $each: reservationDates } } }, 40 { session }); 41 console.log(`${listingsAndReviewsUpdateResults.matchedCount} document(s) found in the listingsAndReviews collection with the name ${nameOfListing}.`); 42 console.log(`${listingsAndReviewsUpdateResults.modifiedCount} document(s) was/were updated to include the reservation dates.`); 43 44 }, transactionOptions); 45 46 if (transactionResults) { 47 console.log("The reservation was successfully created."); 48 } else { 49 console.log("The transaction was intentionally aborted."); 50 } 51 } catch(e){ 52 console.log("The transaction was aborted due to an unexpected error: " + e); 53 } finally { 54 await session.endSession(); 55 } 56 57 }
#Call the Function
Now that we've written a function that creates a reservation using a transaction, let's try it out! Let's create a reservation for Leslie at the Infinite Views listing for the nights of December 31, 2019 and January 1, 2020.
- Inside of
main()
beneath the comment that saysMake the appropriate DB calls
, call yourcreateReservation()
function:
1 await createReservation(client, 2 "leslie@example.com", 3 "Infinite Views", 4 [new Date("2019-12-31"), new Date("2020-01-01")], 5 { pricePerNight: 180, specialRequests: "Late checkout", breakfastIncluded: true });
- Save your file.
- Run your script by executing
node transaction.js
in your shell. - The following output will be displayed in your shell.
1 1 document(s) found in the users collection with the email address leslie@example.com. 2 1 document(s) was/were updated to include the reservation. 3 1 document(s) found in the listingsAndReviews collection with the name Infinite Views. 4 1 document(s) was/were updated to include the reservation dates. 5 The reservation was successfully created.
Leslie's document in the users collection now contains the reservation.
1 { 2 "_id": {"$oid":"5dd68bd03712fe11bebfab0c"}, 3 "email":"leslie@example.com", 4 "name":"Leslie Yepp", 5 "reservations": [ 6 { 7 "name":"Infinite Views", "dates": [ 8 {"$date": {"$numberLong":"1577750400000"}}, 9 {"$date": {"$numberLong":"1577836800000"}} 10 ], 11 "pricePerNight": {"$numberInt":"180"}, 12 "specialRequests": "Late checkout", 13 "breakfastIncluded":true 14 } 15 ] 16 }
The "Infinite Views" listing in the listingsAndReviews
collection now contains the reservation dates.
1 { 2 "_id": {"$oid": "5dbc20f942073d6d4dabd730"}, 3 "name": "Infinite Views", 4 "summary": "Modern home with infinite views from the infinity pool", 5 "property_type": "House", 6 "bedrooms": {"$numberInt":"6"}, 7 "bathrooms": {"$numberDouble":"4.5"}, 8 "beds": {"$numberInt":"8"}, 9 "datesReserved": [ 10 {"$date": {"$numberLong": "1577750400000"}}, 11 {"$date": {"$numberLong": "1577836800000"}} 12 ] 13 }
#Wrapping Up
Today we implemented a multi-document transaction. Transactions are really handy when you need to make changes to more than one document as an all-or-nothing operation.
Be sure you are using the correct read and write concerns when creating a transaction. See the MongoDB documentation for more information.
When you use relational databases, related data is commonly split between different tables in an effort to normalize the data. As a result, transaction usage is fairly common.
When you use MongoDB, data that is accessed together should be stored together. When you model your data this way, you will likely find that you rarely need to use transactions.
This post included many code snippets that built on code written in the first post of this MongoDB and Node.js Quick Start series. To get a full copy of the code used in today's post, visit the Node.js Quick Start GitHub Repo.
Be on the lookout for the next post in this series where we'll discuss change streams.
#Additional Resources
#Series Versions
The examples in this article were created with the following application versions:
Component | Version used |
---|---|
MongoDB | 4.0 |
MongoDB Node.js Driver | 3.3.2 |
Node.js | 10.16.3 |
Questions? Comments? We'd love to connect with you. Join the conversation on the MongoDB Community Forums.