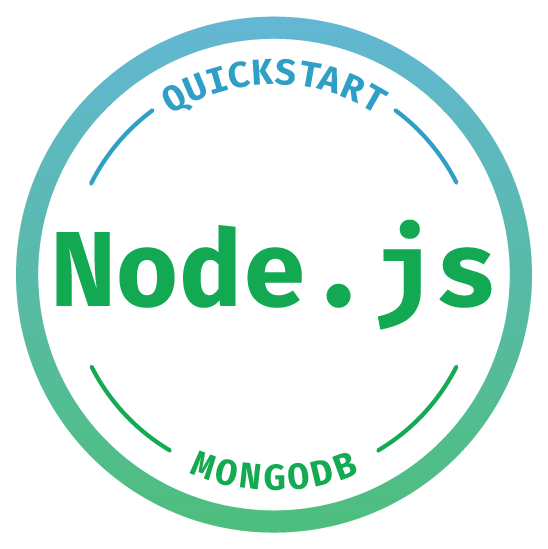
Use Node.js? Want to learn MongoDB? This is the blog series for you!
In this Quick Start series, I'll walk you through the basics of how to get started using MongoDB with Node.js. In today's post, we'll work through connecting to a MongoDB database from a Node.js script, retrieving a list of databases, and printing the results to your console.
#Set up
Before we begin, we need to ensure you've completed a few prerequisite steps.
#Install Node.js
First, make sure you have a supported version of Node.js installed (the MongoDB Node.js Driver requires Node 4.x or greater and for these examples, I've used Node.js 10.16.3).
#Install the MongoDB Node.js Driver
The MongoDB Node.js Driver allows you to easily interact with MongoDB databases from within Node.js applications. You'll need the driver in order to connect to your database and execute the queries described in this Quick Start series.
If you don't have the MongoDB Node.js Driver installed, you can install it with the following command.
1 npm install mongodb
At the time of writing, this installed version 3.3.2 of the driver. Running npm list mongodb will display the currently installed driver version number. For more details on the driver and installation, see the official documentation.
#Create a free MongoDB Atlas cluster and load the sample data
Next, you'll need a MongoDB database. Your database will be stored inside of a cluster. At a high level, a cluster is a set of nodes where copies of your database will be stored.
The easiest way to get started with MongoDB is to use Atlas, MongoDB's fully-managed database-as-a-service. Head over to Atlas and create a new cluster in the free tier. Once your tier is created, load the sample data.
Get started with an M0 cluster on Atlas today. It's free forever, and it's the easiest way to try out the steps in this blog series.
If you're not familiar with how to create a new cluster and load the sample data, check out this video tutorial from MongoDB Developer Advocate Maxime Beugnet.
#Get your cluster's connection info
The final step is to prep your cluster for connection.
In Atlas, navigate to your cluster and click CONNECT. The Cluster Connection Wizard will appear.
The Wizard will prompt you to whitelist your current IP address and create a MongoDB user if you haven't already done so. Be sure to note the username and password you use for the new MongoDB user as you'll need them in a later step.
Next, the Wizard will prompt you to choose a connection method. Select Connect Your Application. When the Wizard prompts you to select your driver version, select Node.js and 3.0 or later. Copy the provided connection string.
For more details on how to access the Connection Wizard and complete the steps described above, see the official documentation.
#Connect to your database from a Node.js application
Now that everything is set up, it's time to code! Let's write a Node.js script that connects to your database and lists the databases in your cluster.
#Import MongoClient
The MongoDB module exports MongoClient
, and that's what we'll use to connect to a MongoDB database. We can use an instance of MongoClient to connect to a cluster, access the database in that cluster, and close the connection to that cluster.
1 const {MongoClient} = require('mongodb');
#Create our main function
Let's create an asynchronous function named main()
where we will connect to our MongoDB cluster, call functions that query our database, and disconnect from our cluster.
The first thing we need to do inside of main()
is create a constant for our connection URI. The connection URI is the connection string you copied in Atlas in the previous section. When you paste the connection string, don't forget to update <username>
and <password>
to be the credentials for the user you created in the previous section. Note: the username and password you provide in the connection string are NOT the same as your Atlas credentials.
1 /**
* Connection URI. Update <username>, <password>, and <your-cluster-url> to reflect your cluster.
* See https://docs.mongodb.com/ecosystem/drivers/node/ for more details
*/ 2 const uri = "mongodb+srv://<username>:<password>@<your-cluster-url>/test?retryWrites=true&w=majority";
Now that we have our URI, we can create an instance of MongoClient.
1 const client = new MongoClient(uri);
Note: When you run this code, you may see DeprecationWarnings around the URL string parser
and the Server Discover and Monitoring engine. If you see these warnings, you can remove them by passing options to the MongoClient. For example, you could instantiate MongoClient by calling new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true })
. See the Node.js MongoDB Driver API documentation for more information on these options.
Now we're ready to use MongoClient to connect to our cluster. client.connect()
will return a promise. We will use the await keyword when we call client.connect()
to indicate that we should block further execution until that operation has completed.
1 await client.connect();
Now we are ready to interact with our database. Let's build a function that prints the names of the databases in this cluster. It's often useful to contain this logic in well named functions in order to improve the readability of your codebase. Throughout this series, we'll create new functions similar to the function we're creating here as we learn how to write different types of queries. For now, let's call a function named listDatabases()
.
1 await listDatabases(client);
Let's wrap our calls to functions that interact with the database in a try/catch
statement so that we handle any unexpected errors.
1 try { 2 await client.connect(); 3 4 await listDatabases(client); 5 6 } catch (e) { 7 console.error(e); 8 }
We want to be sure we close the connection to our cluster, so we'll end our try/catch
with a finally statement.
1 finally { 2 await client.close(); 3 }
Once we have our main()
function written, we need to call it. Let's send the errors to the console.
1 main().catch(console.error);
Putting it all together, our main()
function and our call to it will look something like the following.
1 async function main(){ 2 /**
* Connection URI. Update <username>, <password>, and <your-cluster-url> to reflect your cluster.
* See https://docs.mongodb.com/ecosystem/drivers/node/ for more details
*/ 3 const uri = "mongodb+srv://<username>:<password>@<your-cluster-url>/test?retryWrites=true&w=majority"; 4 5 6 const client = new MongoClient(uri); 7 8 try { 9 // Connect to the MongoDB cluster 10 await client.connect(); 11 12 // Make the appropriate DB calls 13 await listDatabases(client); 14 15 } catch (e) { 16 console.error(e); 17 } finally { 18 await client.close(); 19 } 20 } 21 22 main().catch(console.error);
#List the databases in our cluster
In the previous section, we referenced the listDatabases()
function. Let's implement it!
This function will retrieve a list of databases in our cluster and print the results in the console.
1 async function listDatabases(client){ 2 databasesList = await client.db().admin().listDatabases(); 3 4 console.log("Databases:"); 5 databasesList.databases.forEach(db => console.log(` - ${db.name}`)); 6 };
#Save Your File
You've been implementing a lot of code. Save your changes, and name your file something like connection.js
. To see a copy of the complete file, visit the nodejs-quickstart GitHub repo.
#Execute Your Node.js Script
Now you're ready to test your code! Execute your script by running a command like the following in your terminal: node connection.js
You will see output like the following:
1 Databases: 2 - sample_airbnb 3 - sample_geospatial 4 - sample_mflix 5 - sample_supplies 6 - sample_training 7 - sample_weatherdata 8 - admin 9 - local
#What's next?
Today, you were able to connect to a MongoDB database from a Node.js script, retrieve a list of databases in your cluster, and view the results in your console. Nice!
In future posts in this series, we'll dive into each of the CRUD (create, read, update, and delete) operations as well as topics like change streams, transactions, and the aggregation pipeline, so you'll have the tools you need to successfully interact with data in your databases.
In the meantime, check out the following resources:
#Series versions
This examples in this article were created with the following application versions:
Component | Version used |
---|---|
MongoDB | 4.0 |
MongoDB Node.js Driver | 3.3.2 |
Node.js | 10.16.3 |
Questions? Comments? We'd love to connect with you. Join the conversation on the MongoDB Community Forums.